Salesforce CRM (Apex and Flows)
Send event data from Salesforce CRM (Salesforce Sales Cloud) to Extole using Apex triggers and automate your processes using Flows.
Overview
Integrating Extole and Salesforce CRM (also known as Salesforce Sales Cloud) allows you to set up Apex triggers that correspond to key events in your lead generation program design. When these events occur, you can configure Flows so that the data automatically fires into Extole.
Prerequisites
Requirements | Description |
---|---|
Salesforce Sales Cloud Account and Admin Access | You must have a Salesforce Sales Cloud account and admin-level access, as well as familiarity with the platform, in order to set up this integration. |
Extole Account | You must have an Extole account to leverage this integration. |
Integration
Send Lead Created Events
When leads are created, trigger a RESTful API call to Extole.
Set Up Basic Configuration
You must be a system administrator in order to complete the following steps.
- Navigate to Setup > Remote Site Settings > New and add
<https://api.extole.io
>. - Go to Setup > Apex Classes > New and create an Apex class with an invocable method by copying and pasting the code below.
- Edit the code to query fields on your specific Lead object (line 9), and add those in to be sent to Extole in the request body (line 12)
public with sharing class ExtoleLeadController{
@InvocableMethod
public static void getLeadIds(List<String>leadIds){
makeCallOut(leadIds);
}
@future(callout=true)
public static void makeCallOut(List<String>leadIdList){
List<Lead> leadRec = [SELECT Id LastName,FirstName,Email,Company,AdvocateCode FROM Lead WHERE Id IN:leadIdList];
for(Lead l:leadRec){
String finalBody='{"event_name":"lead_created","data":{'+
'"lead_id":"'+l.Id+'",'+
'"first_name":"'+l.FirstName+'",'+
'"last_name":"'+l.LastName+'",'+
'"email":"'+l.Email+'",'+
'"company":"'+l.Company+'",'+
'"advocate_code":'+l.AdvocateCode+', "app_type":"salesforce_crm"}}';
String endPoint = 'https://api.extole.io/v5/events';
HttpRequest http = new HttpRequest();
http.setEndpoint(endPoint);
http.setMethod('POST');
http.setBody(finalBody);
http.setHeader('Content-Type', 'application/json');
http.setHeader('Authorization', 'Bearer <INSERT TOKEN HERE>');
Http h = new Http();
HttpResponse response = h.send(http);
}
}
}
Automate using Flow on Lead Object
- Go to Setup > Process Automation > Flow > New Flow > Record Triggered Flow > Create.
- Select the Object and configure the Trigger by checking the option
A record is created
. - Choose to optimize the flow for Actions and Related Records.
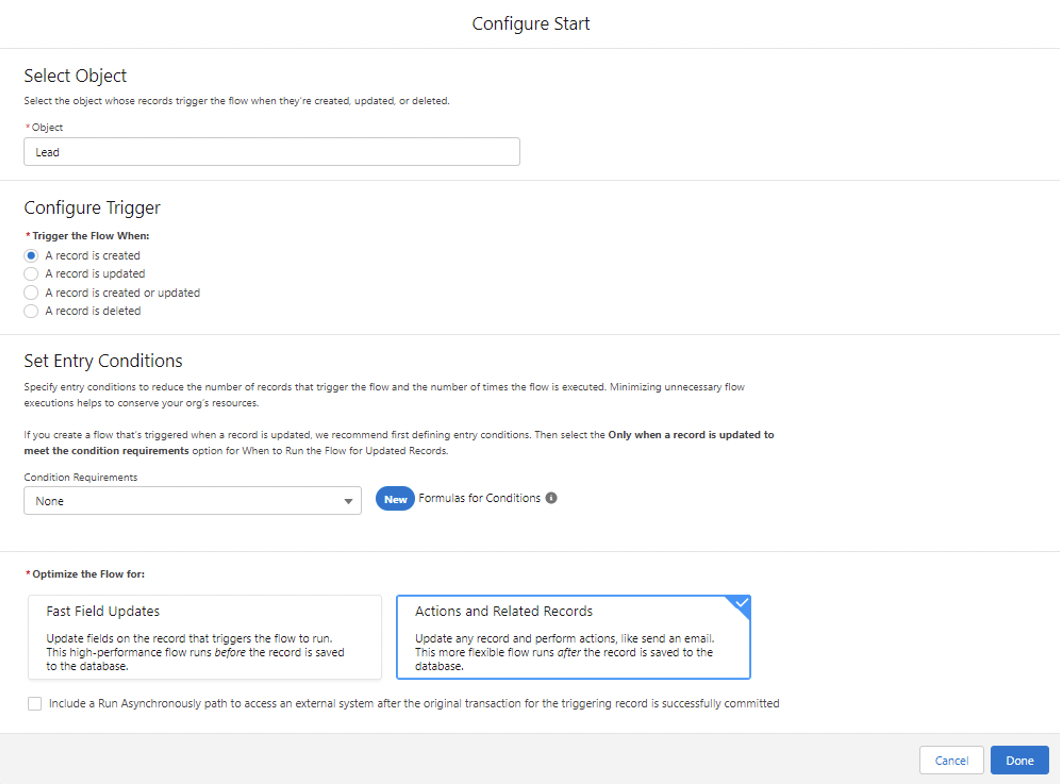
- Click on the + sign to add an element, then select
Action
.
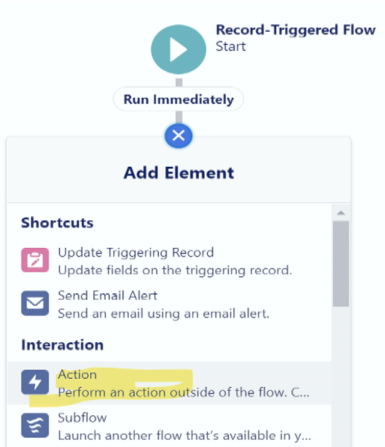
- Select the Apex Action and Controller you just created.
- Select Apex Action and set the input value for
leadId
as{!$Record.Id}
.
- Your final result should look like this:
- Activate the flow and test it by creating the Lead Record.
Send Lead Converted (Opportunity Created) Events
When created leads convert to an opportunity, trigger a RESTful API call to Extole.
Set Up Basic Configuration
You must be a system administrator in order to complete the following steps. The flow will be triggered when the lead will be converted (not on opportunity creation).
- Navigate to Setup > Remote Site Settings > New and add
<https://api.extole.io
>. - Go to Setup > Apex Classes > New and create a new Apex class with an invocable method by copying and pasting the code below.
- Edit the code to query fields on your specific Lead object (line 9), and add those in to be sent to Extole in the request body (line 12)
public with sharing class ExtoleConvertedLeadController{
@InvocableMethod
public static void getLeadIds(List<String>leadIds){
makeCallOutForConvertedLeads(leadIds);
}
@future(callout=true)
public static void makeCallOutForConvertedLeads(List<String>leadIdList){
List<Lead>leadRec = [SELECT Id,LastName,FirstName,Email,AdvocateCode FROM Lead WHERE Id IN:leadIdList];
for(Lead l:leadRec){
String finalBody='{"event_name":"lead_converted","data":{'+
'"lead_id":"'+l.Id+'",'+
'"first_name":"'+l.FirstName+'",'+
'"last_name":"'+l.LastName+'",'+
'"email":"'+l.Email+'",'+
'"advocate_code":"'+l.AdvocateCode+',"app_type":"salesforce_crm"}}';
String endPoint = 'https://api.extole.io/v5/events';
HttpRequest http = new HttpRequest();
http.setEndpoint(endPoint);
http.setMethod('POST');
http.setBody(finalBody);
http.setHeader('Content-Type', 'application/json');
http.setHeader('Authorization', 'Bearer <INSERT TOKEN HERE>');
Http h = new Http();
HttpResponse response = h.send(http);
}
}
}
Important Notes
- This sample code may not reflect your SFDC configuration or custom fields. It may need updating to use fields your team is taking advantage of.
- Feel free to rename the label or variable as per your org coding guidelines.
- The method is tagged with
@InvocableMethod
, which enables this class to be used by Flow.- Keep the auth token in custom metadata or a custom label.
Automate using Flow on Lead Object
For the following steps, you must have System Administrator permission. You must also have a basic understanding of how to create a flow and add filters and actions.
- Go to Setup > Process Automation > Flow > New Flow > Record Triggered Flow > Create.
- Select the Object and configure the Trigger by checking the option
A record is updated
. - Open the Condition Requirements dropdown and select
All Conditions Are Met (AND)
. Set the Field asIsConverted
, the Operator asEquals
, and the Value asTrue
. - Choose to run the flow for updated records every time a record is updated to meet the condition requirements.
- Choose to optimize the flow for Actions and Related Records.
- Click on the + sign to add an element, then select
Action
.
- Select the Apex Action and Controller you just created.
- Select the Apex Action and set the input value for
leadId
as{!$Record.Id}
.
- Your final result should look like this:
- Active the flow and test it by converting the Lead Record.
Send Opportunity Closed / Won Events
When an opportunity is closed or won, trigger a RESTful API call to Extole.
Set Up Basic Configuration
You must be a system administrator in order to complete the following steps.
- Navigate to Setup > Remote Site Settings > New and add
<https://api.extole.io
>. - Go to Setup > Apex Classes > New and create a new Apex class with an invocable method by copying and pasting the following code:
- Edit the code to query fields on your specific Opportunity object (line 9), and add those in to be sent to Extole in the request body (line 12). You may need to use an identifier in the Opportunity object to query a different Object to get the required data. This should happen inside of the for loop on line 11
public with sharing class ExtoleOpportunityController{
@InvocableMethod
public static void getOppoIds(List<String>opportunityId){
makeCallOutForWonOpportunity(opportunityId);
}
@future(callout=true)
public static void makeCallOutForWonOpportunity(List<String>opportunityIdList){
List<Opportunity>oppRec = [SELECT Id,Name,Email FROM Opportunity WHERE Id IN:opportunityIdList];
for(Opportunity op:oppoRec){
String finalBody='{"event_name":"opportunity_closedwon","data":{"lead_id":"123LeadId",'+
'"opportunity_id":"'+op.Id+'",'+
'"first_name":"'+op.Name+'",'+
'"email":'+op.Email+',"app_type":"salesforce_crm"}}';
String endPoint = 'https://api.extole.io/v5/events';
HttpRequest http = new HttpRequest();
http.setEndpoint(endPoint);
http.setMethod('POST');
http.setBody(finalBody);
http.setHeader('Content-Type', 'application/json');
http.setHeader('Authorization', 'Bearer <INSERT TOKEN HERE>');
Http h = new Http();
HttpResponse response = h.send(http);
}
}
}
Important Notes
- This sample code may not reflect your SFDC configuration or custom fields. It may need updating to use fields or objects your team is taking advantage of.
- Feel free to rename the label or variable as per your org coding guidelines.
- The method is tagged with
@InvocableMethod
, which enables this class to be used by Flow.- Keep the auth token in custom metadata or a custom label.
Automate using Flow on Lead Object
For the following steps, you must have System Administrator permission. You must also have a basic understanding of how to create a flow and add filters and actions.
- Go to Setup > Process Automation > Flow > New Flow > Record Triggered Flow > Create.
- Select the Object and configure the Trigger by checking the option
A record is created or updated
. - Open the Condition Requirements dropdown and select
All Conditions Are Met (AND)
. Set the Field asStageName
, the Operator asEquals
, and the Value asClosed Won
. - Choose to run the flow for updated records every time a record is updated to meet the condition requirements.
- Choose to optimize the flow for Actions and Related Records.
- Click on the + sign to add an element, then select
Action
.
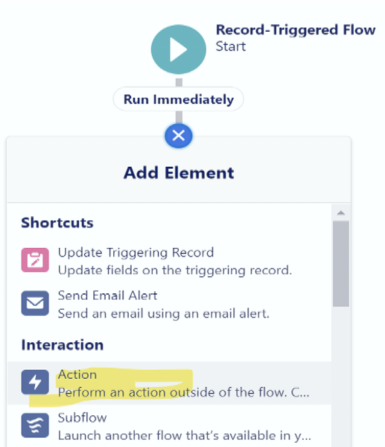
- Select the Apex Action and Controller you just created.
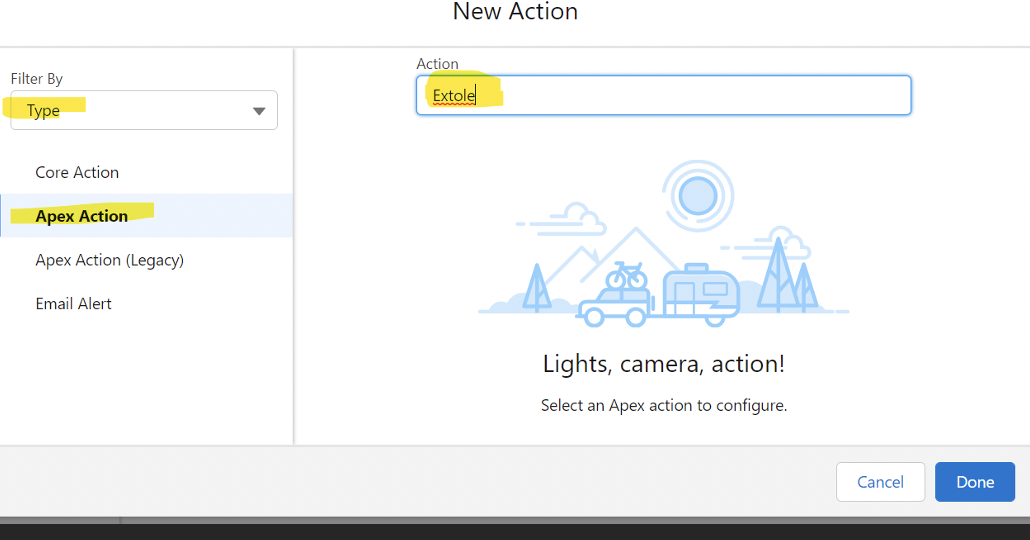
- Select the Apex Action and set the input value for
opportunityId
as{!$Record.Id}
.
- Your final result should look like this:
- Activate and test the flow by changing or creating closed won opportunities.
How-To Guide
Debug Instructions
To debug, you can use the standard debugging options for Salesforce.
- In your Apex code, put
System.debug()
where you would like to debug. - Go to Setup and type Debug Log into the search bar.
- Click on Debug Logs from your search results.
- Click the New button to enable the debug log for a user.
- Select User from the Traced Entity Type dropdown.
- Enter
SFDC_DevConsole
for the Traced Name Entity and Debug Level. - Save the log.
- After setting up the debug log, perform an operation to execute the flow/trigger. For example, you could create a lead, convert a lead, or close an opportunity.
- Executing a flow will generate debug logs. Refresh the debug log page to see them.
- Look for the most recent log and download it to see the response from Extole.
Debug Help
Still need help with debugging? Reference the following Salesforce articles.
Send Additional Salesforce Events to Extole
There are two primary steps that must be set up for an event.
- Create a Service Apex Class that can be used by a flow.
- Create a flow to run for the specified events.
Create Service Apex Controller
You must be a system administrator in order to complete the following steps.
- Navigate to Setup > Remote Site Settings > New and add
<https://api.extole.io
>. - Go to Setup > Apex Classes > New and create a new Apex class with an invocable method by copying and pasting the following code:
public with sharing class ExtoleController {
@InvocableMethod
public static void invokeFromFlow(List<String>recordIds){
makeHttpCallToExtole(recordIds);
}
@future(callout=true)
public static void makeHttpCallToExtole(List<String>recordIds){
List<Opportunity>records = [SELECT Id,Name FROM <OBJECT NAME> WHERE Id IN: recordIds];
for(OBJECT_NAME op: records){
String jsonPayload = '{"<param 1>":"<value_1>","<param 2>":"<value 2>","app_type":"salesforce_crm"}';
String endPoint = 'https://api.extole.io/v5/events';
HttpRequest http = new HttpRequest();
http.setEndpoint(endPoint);
http.setMethod('POST');
http.setBody(finalBody);
http.setHeader('Content-Type', 'application/json');
http.setHeader('Authorization', 'Bearer <INSERT TOKEN HERE>');
Http h = new Http();
HttpResponse response = h.send(http);
}
}
}
Important Notes
- Feel free to rename the label or variable as per your org coding guidelines.
- Replace the object name and update the query as necessary.
- Update the endpoint and other details as necessary.
- Keep the auth token in custom metadata or a custom label.
Create Flow
For the following steps, you must have System Administrator permission. You must also have a basic understanding of how to create a flow and add filters and actions.
- Go to Setup > Process Automation > Flow > New Flow > Record Triggered Flow > Create.
- Select the Object and configure the Trigger. For example, to fire the call when a lead is created, here you would select the Lead object.
- Set the Entry Conditions according to your object and trigger.
- Choose to optimize the flow for Actions and Related Records.
- Click on the + sign to add an element, then select
Action
. - Select whatever Apex Action and controller you want.
- Select Apex Action and set the input value as
{!$Record.Id}
. - Activate the flow and test it by conducting whatever action triggers the event.
Send Additional Data Attributes to Extole
Parameters are passed to Extole's Events API using Apex controllers. Parameters are passed a JSON (key, value pair). You can add as many additional data attributes as you want in the JSON.
Updated 9 months ago